Task automation with node.js using Gulp
Node.js offers significant usefulness in the realm of task automation.
With the help of task runner modules like Grunt or Gulp, developers can automate repetitive tasks, such as minification, concatenation, transpilation, testing, and deployment.
These modules provide a streamlined way to define and execute tasks, reducing manual effort and saving valuable time during the development process.
They offer extensive plugin ecosystems that enable easy integration with various tools and frameworks, allowing developers to tailor their automation workflows to suit their specific project needs.
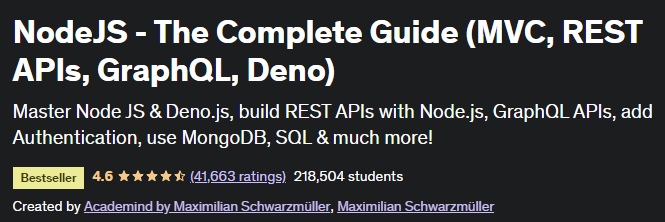
By leveraging Node.js and task runners, developers can achieve increased productivity, maintain code quality, and ensure consistent build processes across projects.
Here’s an example of how to automate a task using Gulp, a popular task runner for Node.js:
const gulp = require('gulp');
const concat = require('gulp-concat');
const uglify = require('gulp-uglify');
const sass = require('gulp-sass');
// Concatenate and minify JavaScript files
gulp.task('scripts', () => {
return gulp
.src('src/js/*.js')
.pipe(concat('bundle.js'))
.pipe(uglify())
.pipe(gulp.dest('dist/js'));
});
// Compile Sass to CSS
gulp.task('styles', () => {
return gulp
.src('src/scss/*.scss')
.pipe(sass().on('error', sass.logError))
.pipe(gulp.dest('dist/css'));
});
// Watch for changes in JavaScript and Sass files
gulp.task('watch', () => {
gulp.watch('src/js/*.js', gulp.series('scripts'));
gulp.watch('src/scss/*.scss', gulp.series('styles'));
});
// Default task: execute 'scripts', 'styles', and 'watch'
gulp.task('default', gulp.parallel('scripts', 'styles', 'watch'));
In this example, we define three tasks using Gulp.
The scripts
task concatenates all JavaScript files, minifies the resulting bundle, and saves it to the dist/js
directory.
The styles
task compiles Sass files to CSS and saves the output to the dist/css
directory.
The watch
task monitors changes in JavaScript and Sass files and triggers the respective tasks whenever a change occurs.
The default
task is executed when running gulp
command without any arguments.
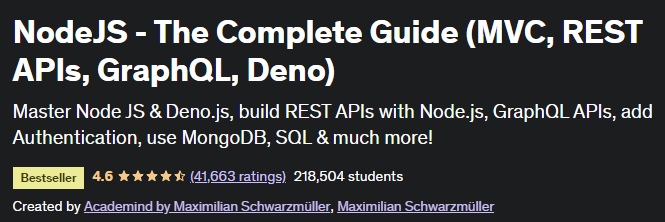
It runs the scripts
, styles
, and watch
tasks in parallel. This setup ensures that changes to JavaScript or Sass files are automatically detected and the appropriate tasks are executed, facilitating a smooth and efficient development workflow.
To run this Gulp automation script, make sure you have Gulp installed globally (npm install -g gulp
) and have the required plugins installed locally (npm install gulp-concat gulp-uglify gulp-sass
).
After that, you can navigate to the project directory and execute the gulp
command to start the automation process.
Learn more IT here
No comments: