How to create a basic HTTP server with Node.js
Node.js is a highly useful technology with a multitude of applications. One of its main advantages is its ability to handle high levels of concurrent connections efficiently.
This makes it particularly well-suited for building scalable and real-time applications, such as chat applications, streaming platforms, and collaborative tools.
Node.js also excels in building APIs and server-side applications, thanks to its lightweight and event-driven architecture.
Additionally, the vast ecosystem of npm (Node Package Manager) modules empowers developers with a wide range of tools and libraries, simplifying the development process and allowing for rapid prototyping and iteration.
Overall, Node.js’s versatility, performance, and rich ecosystem make it a valuable choice for modern web development.
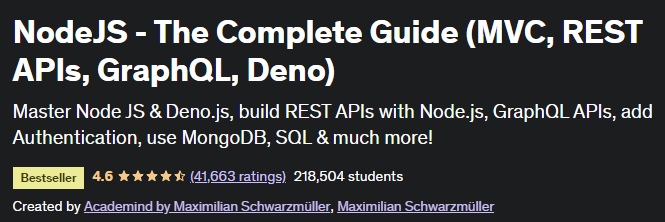
Here’s an example of a complete Node.js program that creates a basic HTTP server:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, world!');
});
const port = 3000;
server.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
In this program, we first import the built-in http
module. We then create an HTTP server using the createServer
method, which takes a callback function as an argument.
The callback function is executed for each incoming request, and it receives the req
(request) and res
(response) objects.
In this example, we set the status code to 200, specify the content type as plain text, and send the response with the message "Hello, world!".
Finally, we specify the port on which the server should listen (in this case, port 3000), and call the listen
method on the server object to start the server.
Once the server is running, it logs a message to the console.
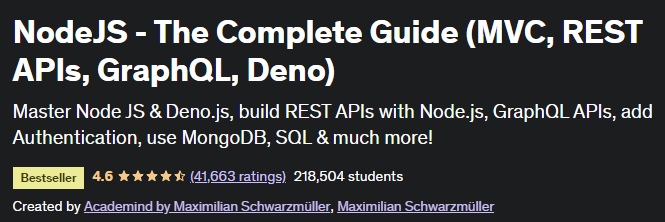
You can run this program using Node.js, and it will create a basic HTTP server that listens on port 3000 and responds with “Hello, world!” for every request.
Learn more IT here
No comments: