React with 3d animation: making a sample 3d animation with React
React with 3D animation combines the power of React’s component-based architecture with the flexibility and immersive experience of 3D animations.
By leveraging libraries like Three.js or React Three Fiber, developers can create interactive and visually appealing 3D animations within their React applications.
React’s declarative syntax and virtual DOM make it easier to manage the state and render updates, while the 3D libraries handle the complexities of rendering and manipulating 3D objects.
This combination allows developers to build rich and interactive user interfaces that bring 3D animations to life.
To create a sample 3D animation with React, you can start by setting up a React project and installing the necessary dependencies.
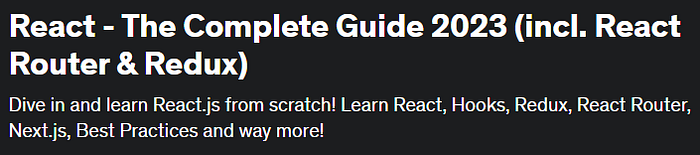
For this example, we’ll use React Three Fiber, a popular library that bridges React and Three.js.
Here’s a sample code snippet that creates a rotating cube:
import React, { useRef } from 'react';
import { Canvas, useFrame } from 'react-three-fiber';
import { Box } from 'drei';
function RotatingCube() {
const cubeRef = useRef();
useFrame(() => {
cubeRef.current.rotation.x += 0.01;
cubeRef.current.rotation.y += 0.01;
});
return (
<Box ref={cubeRef} args={[1, 1, 1]} position={[0, 0, 0]}>
<meshBasicMaterial attach="material" color="blue" />
</Box>
);
}
function App() {
return (
<Canvas>
<ambientLight intensity={0.5} />
<pointLight position={[10, 10, 10]} />
<RotatingCube />
</Canvas>
);
}
export default App;
In this code, we define a RotatingCube
component that uses a useFrame
hook from React Three Fiber to update the rotation of the cube on every frame.
We create a reference using useRef
to access the cube's properties, and then manipulate its rotation in the animation loop.
The App
component sets up the canvas, lighting, and renders the RotatingCube
component.
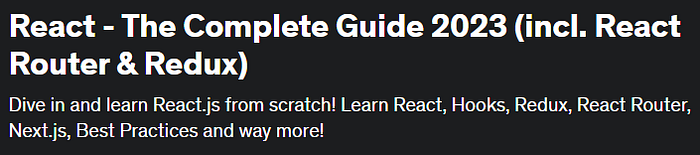
When you run this code, you’ll see a blue cube rotating in 3D space.
You can customize the cube’s size, position, material, and add more complex animations or interactivity as per your requirements.
React Three Fiber provides a powerful set of tools and components for building 3D scenes, making it easier to integrate 3D animations into your React applications.
Comments
Post a Comment